Mastering the Art of Writing Clean and Maintainable Code
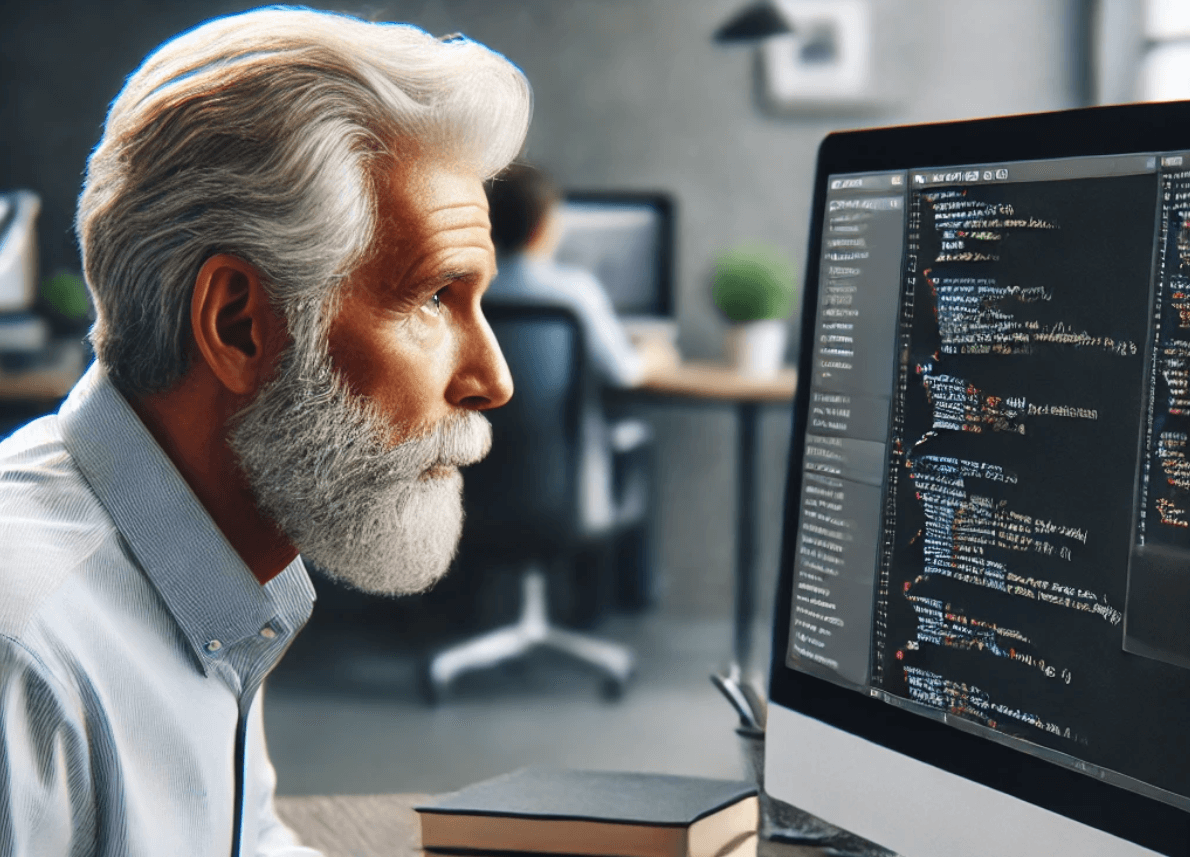
In the world of software development, writing code is just the first step. The true craftsmanship lies in creating code that not only works but is also clean and maintainable. Clean and maintainable code is like a well-structured piece of art, easy to understand, modify, and extend. In this blog post, we will explore the importance of writing clean and maintainable code and provide examples to illustrate best practices.
The Importance of Clean Code
Readability
Clean code is code that is easy to read and understand. When you write code, you're not just communicating with the computer; you're also communicating with other developers (including your future self). Code that is easy to read reduces the cognitive load on developers, making it easier for them to grasp the logic and intent behind the code.
Example of Unclean Code:
x = 10
y = 5
z = x + y
print("The result is: " + str(z))
Example of Clean Code:
first_number = 10
second_number = 5
result = first_number + second_number
print("The result is: " + str(result))
The clean code version uses meaningful variable names, making it clear what the code does.
Maintainability
Maintainable code is code that can be easily modified and extended without introducing bugs or unintended side effects. As software evolves, you'll need to make changes, fix bugs, and add new features. Clean code makes these tasks less error-prone and time-consuming.
Example: Let's say you have a function that calculates the total price of items in a shopping cart:
def calculate_total(cart):
total = 0
for item in cart:
total += item['price'] * item['quantity']
return total
Now, imagine you need to add a discount feature. In clean code, you can extend the function without much hassle:
def calculate_total(cart):
total = 0
for item in cart:
total += item['price'] * item['quantity']
return total
def apply_discount(total, discount_percentage):
discount_amount = total * (discount_percentage / 100)
return total - discount_amount
Collaboration
Clean code promotes collaboration among team members. When everyone follows consistent coding conventions and best practices, it's easier for team members to work together seamlessly. Clean code reduces the friction caused by having to decipher poorly written or inconsistent code.
Best Practices for Writing Clean and Maintainable Code
- Meaningful Variable and Function Names: Use descriptive names that convey the purpose of variables and functions.
- Modularity: Break your code into smaller, reusable modules or functions. This promotes code reusability and makes it easier to test.
- Comments and Documentation: Provide clear comments and documentation to explain complex logic, algorithms, or external dependencies.
- Consistency: Follow a consistent coding style and adhere to coding standards adopted by your team or project.
- Avoid Magic Numbers and Strings: Replace hard-coded values with constants or named variables to improve code readability and maintainability.
- Error Handling: Implement robust error handling to gracefully handle unexpected situations and failures.
- Testing: Write unit tests to ensure that your code functions correctly and consistently. Automated testing helps catch regressions during development.
- Refactoring: Regularly review and refactor your code to eliminate code smells and improve its structure.
Conclusion
Mastering the art of writing clean and maintainable code is a journey that every software developer should embark on. Clean code is not just about aesthetics; it's about improving readability, maintainability, and collaboration. By following best practices and embracing clean code principles, you can create software that stands the test of time and empowers your team to build and maintain exceptional software systems. Happy coding!